How to use Action State
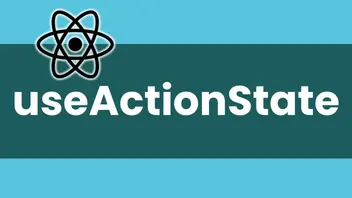
A Guide to useActionState
To complement React Actions React have released a new hook useActionState
that helps manage state, status and visual feedback. These are useful when working with forms.
If you prefer watching rather than reading, check out my video where I go through creating a form in React from scratch and implement useActionState.
This hook simplifies managing form states and form submissions. Using Actions, it captures form input data, handles validation, and error states, reducing the need for custom state management logic. It also exposes a pending
state so you can show a loader while the action is being executed
For example:
"use client"
import { useActionState }
async function incrementCount(prevState: number, formData: FormData) {
return prevState + 1;
}
export default function Counter() {
const [state, formAction, pending] = useActionState(incrementCount, 0);
if (pending) {
return <p>Loading...</p>
}
return (
<form>
<button formAction={formAction}>Increment</button>
<span>{state}</span>
</form>
)
}
The formState
is the value returned by the formAction
.
useActionState()
takes in these 3 parameters:
fn
: The function to be called when the form is submitted or button pressed. It takes the previous state of the form (initially theinitialState
that we pass), followed byFormData
, the argument that aAction
usually receives.initilState
- The value you want the state it to be initiallypermalink (optional)
- A string containing the unique page URL that this form modifies. For use on pages with dynamic content (eg: feeds) in conjunction with progressive enhancement: itfn
is aserverAction
and the form is submitted before the JS bundle loads, the browser will navigate to the specified permalink URL, rather than the current page’s URL.
Publish on 2024-10-22,Update on 2024-11-03